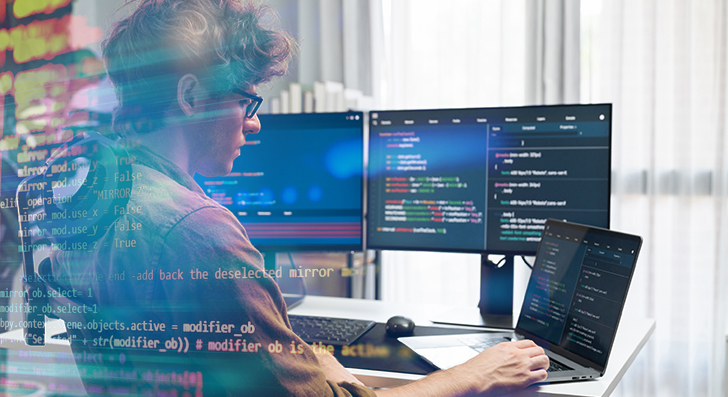
Scalability implies your software can deal with growth—extra people, a lot more information, and much more traffic—without breaking. For a developer, building with scalability in your mind saves time and strain later on. Here’s a transparent and sensible guideline that may help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later on—it should be portion of your system from the start. Numerous apps fail if they develop rapid simply because the first style can’t cope with the additional load. As a developer, you must think early about how your procedure will behave under pressure.
Start off by designing your architecture for being flexible. Prevent monolithic codebases where almost everything is tightly related. Rather, use modular style and design or microservices. These patterns break your application into lesser, impartial sections. Each module or support can scale By itself without the need of affecting The full procedure.
Also, consider your database from working day one. Will it want to manage one million users or perhaps a hundred? Select the suitable style—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t require them but.
One more significant issue is to avoid hardcoding assumptions. Don’t publish code that only will work underneath present-day disorders. Think about what would occur In case your user base doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that support scaling, like information queues or party-pushed devices. These enable your application tackle extra requests without the need of having overloaded.
After you Make with scalability in your mind, you're not just getting ready for success—you might be reducing future problems. A perfectly-prepared program is easier to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the correct Database
Deciding on the appropriate database is a critical Section of developing scalable applications. Not all databases are designed precisely the same, and utilizing the Improper one can gradual you down as well as trigger failures as your application grows.
Commence by comprehending your details. Could it be very structured, like rows inside a desk? If Indeed, a relational databases like PostgreSQL or MySQL is an efficient match. These are sturdy with relationships, transactions, and regularity. They also support scaling tactics like read replicas, indexing, and partitioning to manage much more website traffic and info.
In the event your knowledge is a lot more flexible—like person action logs, products catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured data and may scale horizontally extra effortlessly.
Also, consider your read through and generate patterns. Do you think you're doing numerous reads with much less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Look into databases that could tackle higher publish throughput, or maybe event-primarily based info storage devices like Apache Kafka (for non permanent data streams).
It’s also clever to think ahead. You may not want Innovative scaling features now, but choosing a database that supports them implies you gained’t need to have to change later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your knowledge determined by your entry designs. And generally watch databases effectiveness when you develop.
In brief, the correct database depends upon your app’s structure, velocity requires, And exactly how you hope it to mature. Choose time to pick sensibly—it’ll help you save many issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, just about every smaller delay provides up. Badly created code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s crucial that you Construct effective logic from the start.
Get started by producing clear, easy code. Stay away from repeating logic and remove just about anything unwanted. Don’t select the most complex Alternative if an easy 1 works. Maintain your functions shorter, centered, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take as well very long to run or takes advantage of excessive memory.
Subsequent, evaluate your databases queries. These frequently gradual issues down much more than the code itself. Be certain Each and every question only asks for the data you click here truly require. Prevent Choose *, which fetches everything, and as a substitute select distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, In particular throughout huge tables.
For those who discover precisely the same knowledge remaining requested over and over, use caching. Store the outcomes briefly applying resources like Redis or Memcached and that means you don’t really have to repeat costly operations.
Also, batch your databases functions whenever you can. In place of updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your app far more economical.
Remember to test with huge datasets. Code and queries that operate high-quality with a hundred records could crash every time they have to handle 1 million.
In short, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques assistance your software continue to be sleek and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more consumers and a lot more targeted traffic. If anything goes by just one server, it will eventually immediately turn into a bottleneck. That’s where load balancing and caching come in. Both of these applications enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server undertaking every one of the do the job, the load balancer routes people to diverse servers depending on availability. This implies no one server receives overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information temporarily so it might be reused promptly. When consumers ask for the exact same details again—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You are able to serve it within the cache.
There are 2 common forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores facts in memory for quick obtain.
2. Customer-side caching (like browser caching or CDN caching) outlets static files near to the user.
Caching cuts down database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve usually. And generally make certain your cache is up-to-date when data does adjust.
To put it briefly, load balancing and caching are uncomplicated but potent instruments. Together, they help your application tackle much more people, stay quickly, and Get well from complications. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To make scalable programs, you require applications that let your app expand simply. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess foreseeable future ability. When website traffic boosts, you could increase more resources with just a few clicks or automatically utilizing auto-scaling. When visitors drops, you are able to scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and safety resources. You'll be able to give attention to developing your app instead of running infrastructure.
Containers are A different critical Resource. A container deals your app and everything it needs to operate—code, libraries, options—into one particular unit. This makes it quick to maneuver your app in between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If just one portion of one's application crashes, it restarts it instantly.
Containers also make it straightforward to independent aspects of your app into services. You may update or scale elements independently, which happens to be great for performance and dependability.
In brief, working with cloud and container resources usually means you may scale quick, deploy conveniently, and Recuperate promptly when issues transpire. If you'd like your application to grow with no limits, commence applying these resources early. They help save time, reduce threat, and assist you remain focused on setting up, not fixing.
Observe Every thing
In case you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is executing, place challenges early, and make much better choices as your app grows. It’s a vital part of creating scalable programs.
Get started by monitoring standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this details.
Don’t just monitor your servers—keep track of your app also. Keep watch over just how long it requires for end users to load web pages, how often problems come about, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s happening inside your code.
Set up alerts for important problems. For example, if your reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified right away. This aids you repair problems fast, normally right before people even observe.
Monitoring can also be practical when you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again before it results in true harm.
As your application grows, targeted traffic and info increase. Devoid of monitoring, you’ll miss indications of difficulty right until it’s way too late. But with the proper applications in position, you stay on top of things.
In short, checking aids you keep the app reliable and scalable. It’s not almost recognizing failures—it’s about comprehension your method and making certain it works properly, even under pressure.
Ultimate Views
Scalability isn’t just for significant organizations. Even compact apps will need a strong foundation. By building very carefully, optimizing sensibly, and using the appropriate tools, it is possible to build applications that grow easily without the need of breaking under pressure. Start off small, Feel major, and build sensible.